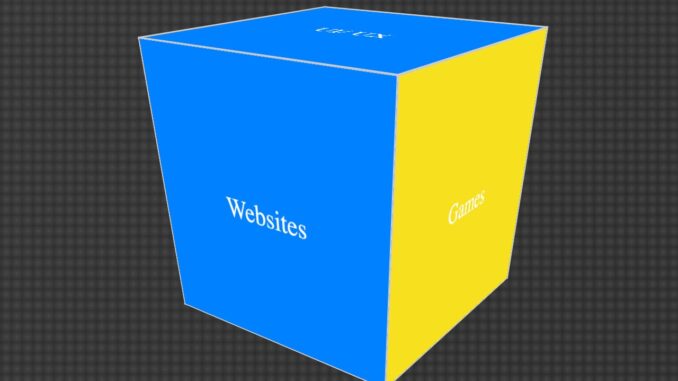
Last Updated on May 2, 2025 by E. Scott
In this blog post, we’ll explore how to create a visually appealing 3D rotating cube using a CSS3 animation. This effect can be used to add a dynamic touch to any web page, making it more engaging and interactive. We’ll break down the CSS step by step, explaining each part and how it contributes to the final result.
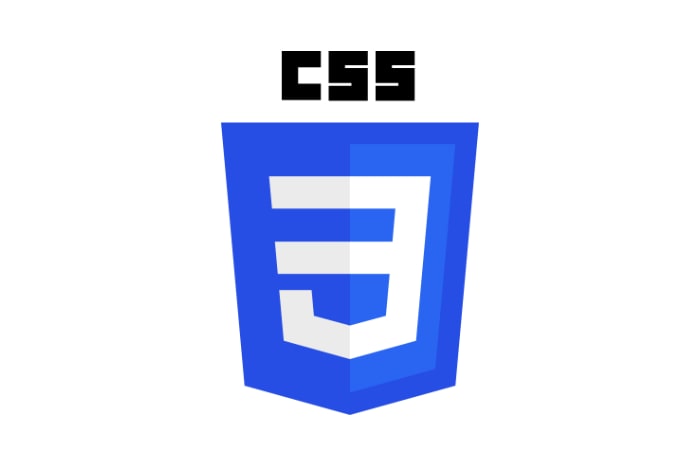
Table of Contents
This is the basic HTML structure of the CSS3 animation.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>3D Rotating Cube</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<main>
<div class="scene">
<div class="cube">
<div class="cube__face cube__face--front">Front</div>
<div class="cube__face cube__face--back">Back</div>
<div class="cube__face cube__face--right">Right</div>
<div class="cube__face cube__face--left">Left</div>
<div class="cube__face cube__face--top">Top</div>
<div class="cube__face cube__face--bottom">Bottom</div>
</div>
</div>
</main>
</body>
</html>
CSS3 Animation
Now let’s look at the core of what powers the cube. This should all look very familiar.
html, body, main {
height: 100%;
}
main {
display: flex;
}
body {
margin: 0;
justify-content: center;
align-items: center;
background: linear-gradient(135deg, #1e1e1e, #343434, #1e1e1e);
background-size: 300% 300%;
animation: gradientAnimation 15s ease infinite;
position: relative;
overflow: hidden;
}
- html, body, main: These elements are set to have a height of 100% to ensure they take up the full viewport height.
- main: Using display: flex; centers the content both horizontally and vertically.
- body: This sets the margin to 0 to remove default browser margins. The justify-content and align-items properties center the content. The background is a linear gradient that animates to create a dynamic effect.
This is very subtle gradient CSS3 animation:
@keyframes gradientAnimation {
0% { background-position: 0% 50%; }
50% { background-position: 100% 50%; }
100% { background-position: 0% 50%; }
}
@keyframes gradientAnimation: This CSS3 animation changes the background position of the gradient, creating a smooth transition effect.
Followed by the textured overlay.
body::before {
content: '';
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: radial-gradient(circle at 50% 50%, rgba(255, 255, 255, 0.1), rgba(0, 0, 0, 0.1)), radial-gradient(circle at 50% 50%, rgba(255, 255, 255, 0.1), rgba(0, 0, 0, 0.1));
background-size: 10px 10px;
opacity: 0.5;
z-index: -1;
}
body::before: This pseudo-element adds a textured overlay to the background. The radial-gradient creates a subtle pattern, and the opacity makes it semi-transparent.
3D Scene and the Cube
.scene {
width: 200px;
perspective: 600px;
margin: 100px auto;
}
.cube {
width: 100%;
height: 100%;
position: relative;
transform-style: preserve-3d;
animation: cubeRotation 7s infinite linear;
}
- .scene: This container sets the perspective for the 3D effect and centers the cube.
- .cube: The cube itself is set to be a 3D object with transform-style: preserve-3d;.
This next part of the CSS3 animation is responsible for defining the individual faces of the 3D cube and animating its rotation. Let’s break it down step by step:
Defining the Cube Faces
Each face of the cube is defined with a specific background color and transformation to position it correctly in 3D space.
.cube__face {
position: absolute;
width: 200px;
height: 200px;
border: 1px solid #ccc;
text-align: center;
line-height: 200px;
font-size: 20px;
color: #ffffff;
}
.cube__face: This is the base class for all cube faces. It sets the face to be an absolute positioned element with a fixed size, border, text alignment, line height, font size, and text color.
Individual Face Styling
Each face of the cube is styled with a specific background color and transformation to position it correctly in 3D space.
.cube__face--front {
background-color: #0080ff;
transform: rotateY(0deg) translateZ(100px);
}
.cube__face--back {
background-color: #007acc;
transform: rotateY(180deg) translateZ(100px);
}
.cube__face--right {
background-color: #f7df1e;
transform: rotateY(90deg) translateZ(100px);
}
.cube__face--left {
background-color: #1e1e1e;
transform: rotateY(-90deg) translateZ(100px);
}
.cube__face--top {
background-color: #0080ff;
transform: rotateX(90deg) translateZ(100px);
}
.cube__face--bottom {
background-color: #007acc;
transform: rotateX(-90deg) translateZ(100px);
}
- .cube__face–front: The front face is positioned by rotating it 0 degrees around the Y-axis and translating it 100px along the Z-axis.
- .cube__face–back: The back face is positioned by rotating it 180 degrees around the Y-axis and translating it 100px along the Z-axis.
- .cube__face–right: The right face is positioned by rotating it 90 degrees around the Y-axis and translating it 100px along the Z-axis.
- .cube__face–left: The left face is positioned by rotating it -90 degrees around the Y-axis and translating it 100px along the Z-axis.
- .cube__face–top: The top face is positioned by rotating it 90 degrees around the X-axis and translating it 100px along the Z-axis.
- .cube__face–bottom: The bottom face is positioned by rotating it -90 degrees around the X-axis and translating it 100px along the Z-axis.
Cube Rotation Animation
The cube is animated to rotate continuously using a keyframes animation.
@keyframes cubeRotation {
from {
transform: rotateX(0deg) rotateY(0deg);
}
to {
transform: rotateX(360deg) rotateY(360deg);
}
}
@keyframes cubeRotation: This defines the animation for the cube. The from state sets the initial rotation to 0 degrees on both the X and Y axes. The to state sets the final rotation to 360 degrees on both the X and Y axes, creating a full rotation.
Applying the CSS3 Animation
The animation is applied to the cube using the animation property.
.cube {
width: 100%;
height: 100%;
position: relative;
transform-style: preserve-3d;
animation: cubeRotation 7s infinite linear;
}
.cube: The animation property is set to cubeRotation 7s infinite linear, which means the cube will rotate continuously for 7 seconds, and the rotation will be linear (constant speed).
Conclusion
- Cube Faces: Each face of the cube is positioned using 3D transformations to create the 3D structure.
- Animation: The cube is animated to rotate continuously, giving the appearance of a 3D object in motion.
The document titled Beautiful Cube CSS3 Animation in 3D Space demonstrates the application of CSS3 animation to create a visually engaging 3D rotating cube effect. By utilizing keyframes and CSS properties like transform-style and animation, the cube rotates continuously for 7 seconds with a linear speed, providing users with an immersive visual experience. It is important to note that CSS3 animations can be resource-intensive due to the processing power required.
Leave a Reply