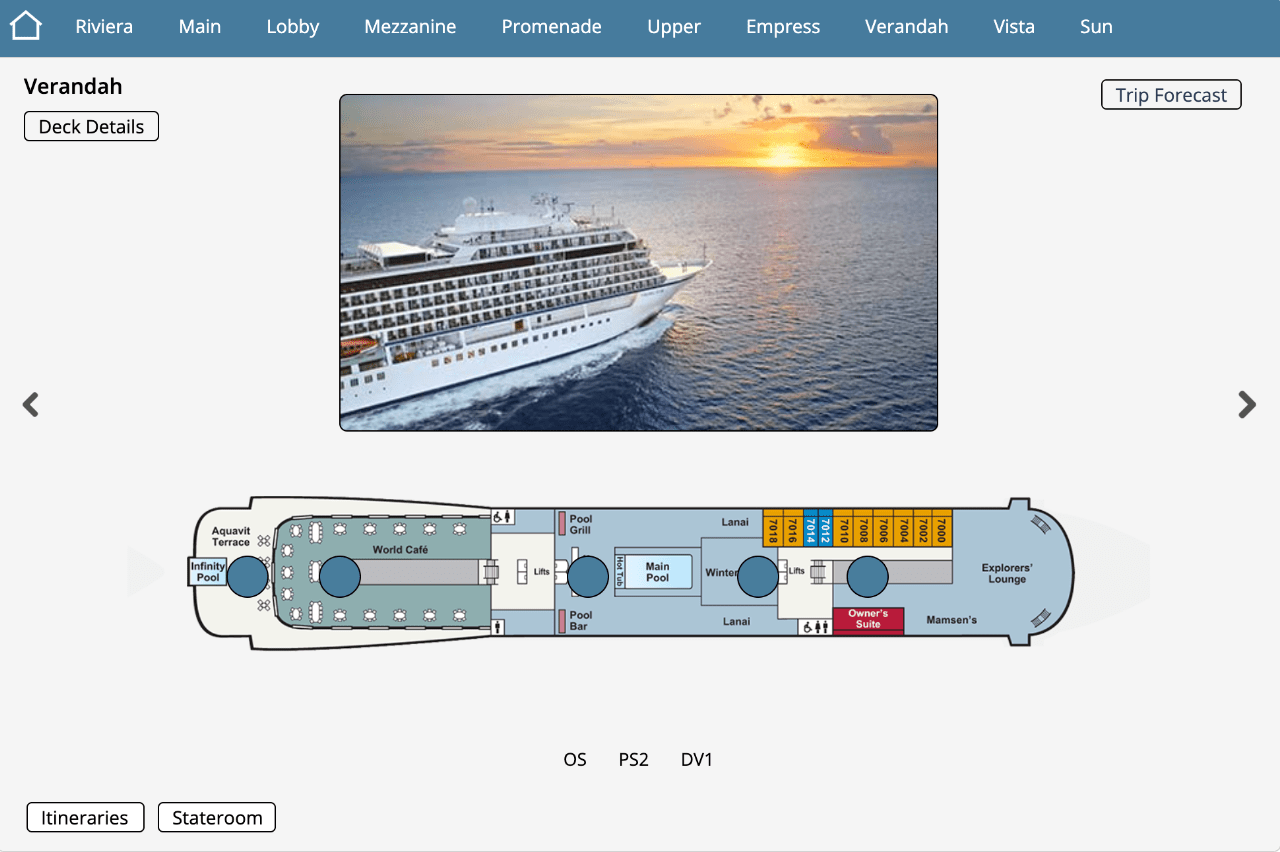
Last Updated on June 29, 2024 by E. Scott
I designed and developed this AngularJS project way back in 2017 for Viking Cruises. They wanted something interactive and innovative. Something they could add to their website as a standalone application. At the time, the only way consumers could explore the decks was by downloading a PDF. I sought out to do more. In turn, bringing a better user experience to the client. View the working demo, or explore the code on CodePen.
Table of Contents
AngularJS Project Directives
Being pressed for time, and without the leisure to create and revise a mock, I simply used one file for numerous controllers. Loading was taken care of quite well with a custom loader. I created an image and video gallery template with a custom directive. These are the five directives I created. The last three are for image galleries and the YouTube player. There’s 10 pages with image galleries. Each page has 3-5 image galleries. So there’s 30-50 image galleries all powered by the same directive/ template.
In Angular 2+, directives are quite different. There’s component directives. Structural and attribute directives. As a whole, they’re known as classes used for adding new behavior to template elements. More specifically, they’re functions found by the compiler used to extend the wherewithal of HTML. Think of them as modifiers. Beyond the three types of directives, we can also create our own.
angular.module("vikingApp").directive("itineraries", function ($http) {
return {
// ITINERARIES SLIDER TEMPLATE
templateUrl: "pages/itineraries.html",
restrict: "E",
link: function (scope, element, attrs) {
// LOAD JSON
$http.get("app/itineraries.json").then(function (myData) {
$.ajax({ cache: false }); // IE & EDGE FIX
scope.trips = myData.data;
});
scope.trips = [];
scope.value = 0;
scope.nextTrip = function () {
scope.value++;
if (scope.value > scope.trips.length - 1) {
scope.value = 0;
}
};
scope.prevTrip = function () {
scope.value--;
if (scope.value < 0) {
scope.value = scope.trips.length - 1;
}
};
}
};
});
// ITINERARIES GRID TEMPLATE
angular.module("vikingApp").directive("gridView", function ($http) {
return {
templateUrl: "pages/itinerariesGrid.html",
restrict: "E",
link: function (scope, element, attrs) {
// FILTER TABLE
scope.trips = [];
scope.sortType = "days";
scope.sortReverse = false;
scope.searchTable = "";
}
};
});
// IMAGE GALLERY TEMPLATE
angular.module("vikingApp").directive("gallery", function () {
return {
templateUrl: "pages/imageGallery.html",
restrict: "E",
link: function (scope, element, attrs) {
scope.changeImg = function (event) {
event.event || window.event;
var targetEvent = event.target || window.event;
if ((targetEvent.tagName = "IMG")) {
mainImg.src = targetEvent.getAttribute("src");
}
};
}
};
});
// IMAGE GALLERY TEMPLATE
angular.module("vikingApp").directive("discoverShip", function (divService) {
return {
templateUrl: "pages/discoverShip.html",
restrict: "E",
link: function (scope, element, attrs) { }
};
});
// VIDEO PLAYER
vikingApp.directive("myYoutube", function ($sce) {
return {
restrict: "EA",
scope: { code: "=" },
replace: true,
template:
'<div><iframe src="{{url}}" frameborder="0" allowfullscreen></iframe></div>',
link: function (scope) {
scope.$watch("code", function (newVal) {
if (newVal) {
scope.url = $sce.trustAsResourceUrl(
"https://www.youtube.com/embed/" + newVal
);
}
});
}
};
});
Final Thoughts
Regardless of whether the project is an archaic or thriving framework, it’s the architecture that matters. How it’s built. How the features are divided up. Harnessing templates, properly sharing data, and loading assets for instance are of upmost importance. Yes, many of the frameworks of yesterday and today innately advocate these developmental principles, but not everyone takes advantage of them.
Just recently for instance, I discovered a coworker trying to query the DOM via getElementById, in an Angular micro frontend. He had no idea what life cycle hooks are. He was unaware of and Renderer2. I’ve seen the same function duplicated across numerous components instead of using a service. Senior devs that don’t know how to use observables in TypeScript. But I digress. I hope you’ve enjoyed this brief explanation and check out my AngularJS project!
Leave a Reply