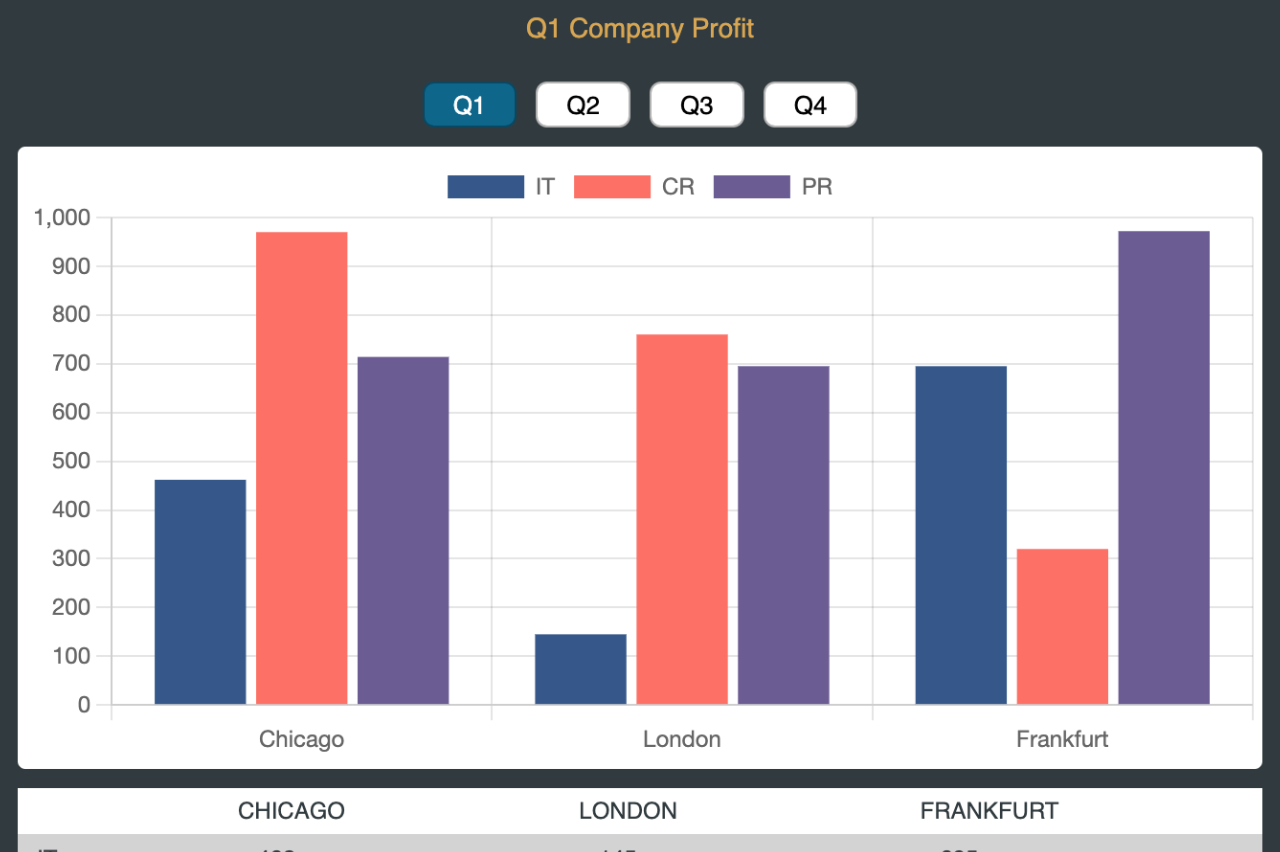
Over the years I’ve built numerous Chartjs bar charts. Used in analytics, data driven UIs, server up time, etc. This time however I decided to build one just for fun. This chartjs bar chart shows mock quarterly data via JSON. Each button loads new data indicative of a payload. Data is accessible by hovering over each bar and displayed in the table below. Similarly, I built this custom line chart.
The code is simple too. The data interface mocks each bar as shown below.
export interface BarInterface {
lable: string;
data: Array<number>;
backgroundColor: string;
}
Data is not complex whatsoever. Take a look at this sample shown below. You can even make changes and see the results take effect immediately of course. Not too bad, right?
[
{
"label": "IT",
"data": [62, 145, 95],
"backgroundColor": "#34568B"
},
{
"label": "CR",
"data": [70, 60, 20],
"backgroundColor": "#FF6F61"
},
{
"label": "PR",
"data": [714, 695, 972],
"backgroundColor": "#6B5B95"
},
{
"label": "ROI",
"data": [200, 90, 150],
"backgroundColor": "#88B04B"
}
]
In the actual class, I declare the variables. Then in OnInit, initialize the canvas, load the data, and let ChartJS work its magic. This is accomplished by configuring the options. Responsiveness, legend, padding, axes, and type. loadData populates the data array with the appropriate values. Then there’s a render method that rerenders the graph when it’s resized. I believe the only other thing that was done was changing a setting in tsconfig.json (“resolveJsonModule”: true). Something which I don’t believe is necessary in later versions. But just an FYI.
import { Component, OnInit } from '@angular/core';
import { Chart } from 'chart.js';
import { BarInterface } from './data.interface';
import * as chartData from './data.json';
import * as q2 from './q2.json';
import * as q3 from './q3.json';
import * as q4 from './q4.json';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
host: {
'(window:resize)': 'onResize($event)',
},
})
export class AppComponent implements OnInit {
dataArray: BarInterface[] = [];
data: any;
chart: any;
quarter: string = 'Q1';
q1: any = chartData;
q2: any = q2;
q3: any = q3;
q4: any = q4;
ngOnInit() {
let options: any,
ctx: any = document.getElementById('areaChart') as HTMLElement;
ctx.style.backgroundColor = '#FFFFFF';
this.loadData(null, this.quarter);
this.data = {
labels: ['Chicago', 'London', 'Frankfurt'],
datasets: this.dataArray,
};
options = {
responsive: false,
legend: {
display: false,
},
layout: {
padding: 15,
},
scales: {
xAxes: [
{
stacked: true,
gridLines: {
display: true,
},
ticks: {
display: true,
fontSize: 12,
},
},
],
yAxes: [
{
stacked: true,
gridLines: {
display: true,
},
ticks: {
display: true,
fontSize: 12,
},
},
],
},
};
this.chart = new Chart(ctx, {
type: 'bar',
data: this.data,
options: options,
});
}
loadData(arr: any, span: any) {
this.dataArray = [];
this.quarter = span;
arr === null ? (arr = chartData) : '';
for (let key in arr) {
if (arr.hasOwnProperty(key)) {
this.dataArray.push(arr[key]);
}
}
if (arr != null && this.data != undefined) {
this.data.datasets = this.dataArray;
this.chart.update();
}
}
// Refresh canvas dimensions
onResize(event: any) {
this.chart.render();
}
}
Note however. This has not been updated to version 3.0 and will not work in later versions of Angular. Sorry, I haven’t had the chance! Realistically, my posts are meant for learning and ideation vs copy-paste. Though I welcome either. V2 or V3—chartjs is an amazing library and one in which I’ve really enjoyed using. Once you wrap your head around the concepts, it’s a pleasure to use in a most applications. I’ve used this in both Angular, vanilla JS, and React. For a more interactive project sample, check out this one.
To see more fully developed projects similar to this one, visit my projects page. Anywho. I hope you find this useful, can implement it, or simply think it’s educational. Regardless, please see a working version of Chartjs Bar Chart UI on Stackblitz here.
Leave a Reply