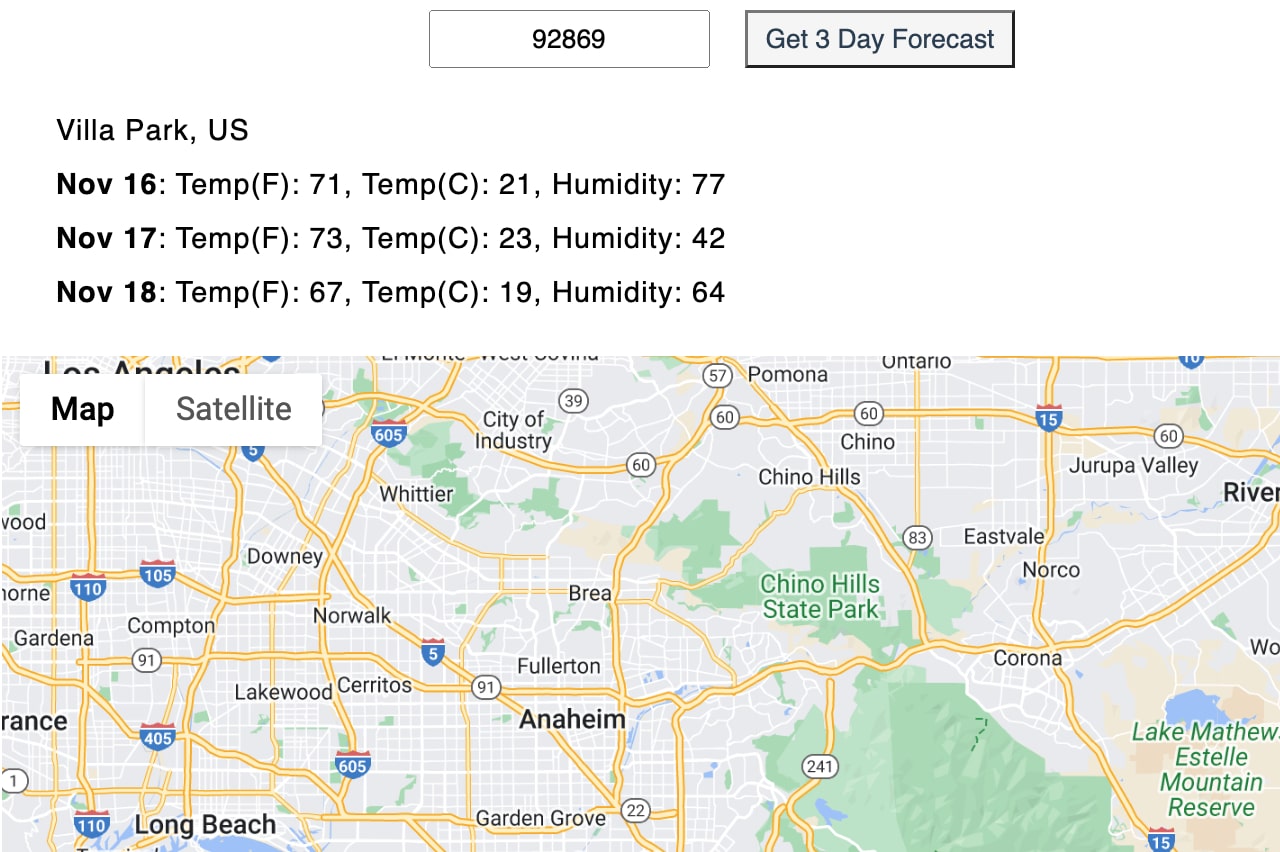
I love this Open Weather Map API. So much can be done with the payload. I created the app below a while ago. As you may have noticed the jQuery. It’s not extensive. But to the trained eye, one can catch the $(…). I digress. jQuery, JavaScript, or TypeScript. This is a neat data driven UI that returns the weather and plots the coordinates on a Google Map. The longitude and latitude are returned from the entered city or zip code. In recent years however, Google changed their maps API so it’s a little more complex to use.
Regardless, let’s jump right in to it. The below code is the all the HTML that’s needed. The getForecast function does it all.
<div class="forecastAPI">
<input id="sevenDay" type="text" placeholder="Enter City Destination">
<button type="buton" onclick="getForecast()" class="apiBtn">Get 3 Day Forecast</button>
<div id="multiDay"></div>
<div id="maps"></div>
</div>
In this block, I create the date object. This is used in the next code block to display the results for the multi day selection.
var myDate = new Date(),
time = myDate.toLocaleTimeString(),
month = myDate.getMonth(),
months = [ "Jan", "Feb", "March", "April", "May", "June", "July", "Aug", "Sept", "Oct", "Nov", "Dec" ],
today = myDate.getDate(),
year = myDate.getFullYear();
Usually I’d break this up. As it’s a bit messy. But, it’s a CodePen I did for fun a while back. Below, the form field value is captured and then used in the URL as a parameter. If you decide to use this code sample, please get your own API key here.
See the Pen Openweather Map Demo by East Coast Developer (@eastcoastdeveloper) on CodePen.
Thereafter, a callback is being used to plot the map location. Lon references the longitude and lat, the latitude. I then get the map element, plot the coordinates, set the zoom, and a few additional options. The for loop at the bottom is to display the three days. The bizarre numeric formula is to convert the number to celsius so both formats are displayed. See it in action here.
/* MULTIPLE DAYS */
function getForecast() {
var cityID = $("#sevenDay").val(),
multiDay = document.getElementById("multiDay"),
multipleDay = "https://api.openweathermap.org/data/2.5/forecast/daily?q=" + cityID + "&appid=409cc3736e4fa7176890bed5e9656117";
$.getJSON(multipleDay, weatherCallback);
function weatherCallback(forecast) {
var lon = forecast.city.coord.lon,
lat = forecast.city.coord.lat,
myMap = document.getElementById('maps'),
mapOptions = {
center: new google.maps.LatLng(lat, lon),
zoom: 10,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
var map = new google.maps.Map(document.getElementById("maps"), mapOptions);
myMap.style.display = "block";
multiDay.innerHTML = forecast.city.name + ", " + forecast.city.country + "<br>";
for (var i = 0; i < 3; i++) {
multiDay.innerHTML += "<b>" +
months[month] + " " + (today + i) + "</b>: Temp(F): " +
(Math.ceil((forecast.list[i].temp.max - 273.15) * 1.8) + 32) +
", Temp(C): " + Math.ceil((forecast.list[i].temp.max - 273.15).toFixed(0)) +
", Humidity: " + forecast.list[i].humidity + "<br>";
}
}
}
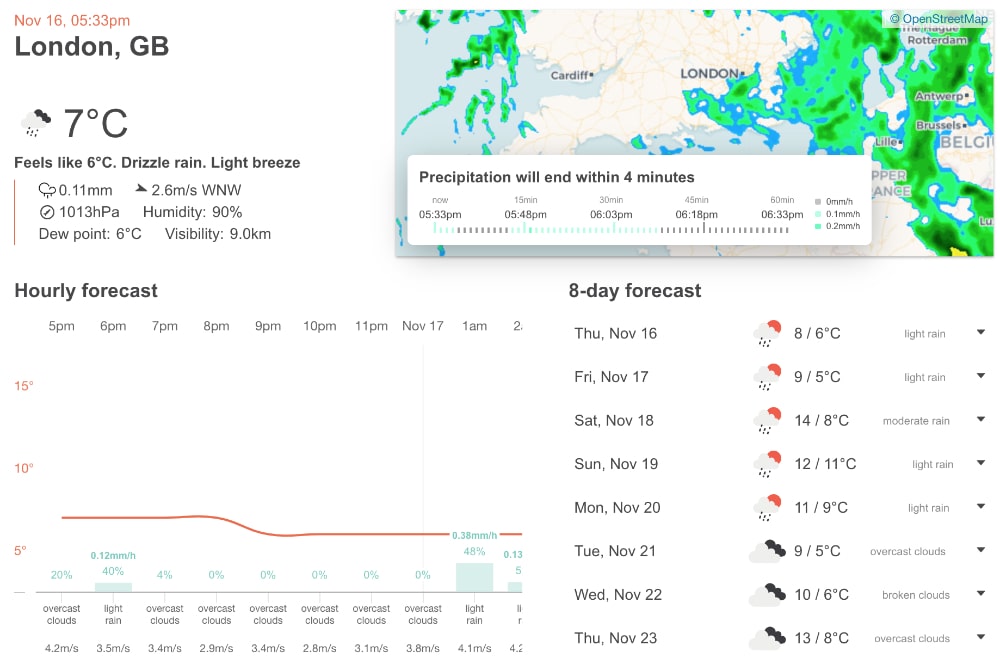
The above picture is from Open Weather Map showing the potential UI we could build using their payload. Definitely similar to the one I’ve built. To see other projects similar to this one, see the full list here. Relative to API projects, check out the OMDB API or the NASA API.
I’ve used this a number of times in different projects and I always enjoy it. Regardless of the technology being used it’s a fun and fast feature. Ideal for travel websites and the like. Have you ever built something like this? Do share in the comments below! Nevertheless, I hope you’ve found this useful. If you’ve never used it, check out the Open Weather Map API.
Leave a Reply