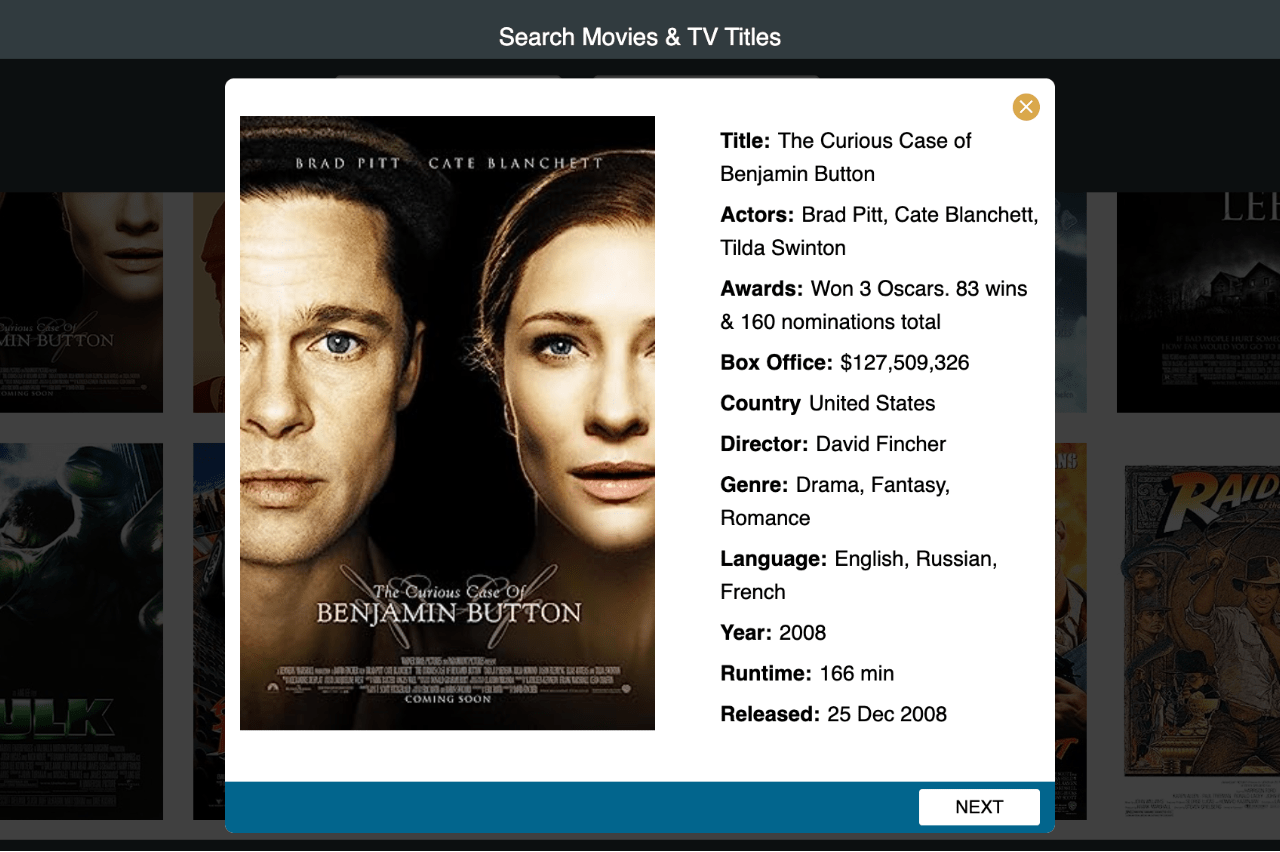
This OMDB API is an example of a data driven UI. Other API related projects are the NASA API and the REST Countries API. Or see a detailed list of frontend dev projects here.
Enter a movie or TV show (must be exact) and watch the results populate the UI. By means of OMDB’s endpoint, we’re fetching data, and populating it accordingly. Though this could no doubt be accomplished in React, Vue, or with vanilla JavaScript, this is an Angular app. If you’re familiar with TypeScript, it should be no problem to convert. Toggle between poster and table layout to get the full scope of the interface.
Upon entering either only a title or both fields, pressing return clears thereof and searches. Table view is the default. Slide to the left to show numerous other fields such as director, genre, language, year, and more. This entire section could be modified slightly to sort columns. This feature however is not currently developed. Regardless, the total number of queries is shown at bottom. Search and footer are fixed.
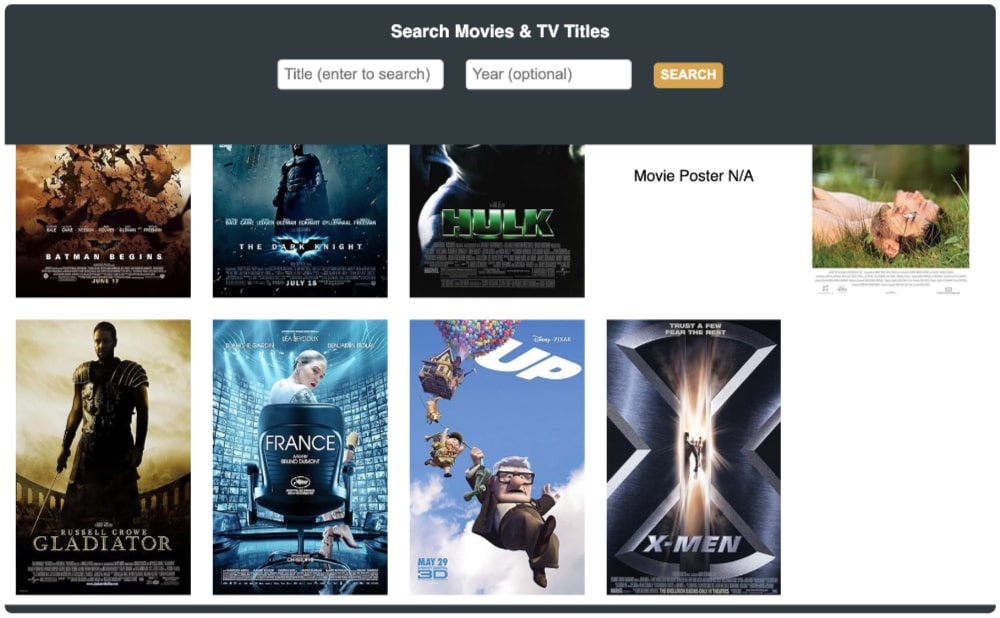
Toggling views snaps to movie posters. Each of which neatly stack as more results come in.
Selecting an item opens a preview as expected accompanied with a close, and navigation buttons. All of which are fully responsive.
All you really need to run this is an API key. Get yours here, then simply plug it into the environments file. Customize it however you see fit. Leave a comment below with your app. I’d love to see what you did with the OMDB api.
My favorite part about building this was how easy it was to use the API. It gives us (the developers) full control over the experience. Data can be fetched with just a title, or returned by year and episode.
Leave a Reply